You might wonder: why would you even want to do this? Well, in some cases I need to have a randomized data set for testing or education purposes. Postman is a tool that I use on an almost daily basis, so that’s an obvious choice for me.
The scenario
In this case, I just wanted to easily test an Azure API Management policy that emits custom metrics to Application Insights. I’ve created a Order API, that processes simple Orders. The content of these orders should be randomized in a controlled manner.
{ "date": "2021-12-16T14:50:37.374Z", "customer": "Your Azure Coach", "type": "External", "department": "Sports", "items": [ { "product": "Hat", "amount": 2 }, { "product": "Bacon", "amount": 55 }, { "product": "Chips", "amount": 27 } ] }
The solution
Dynamic variables
Postman comes out-of-the-box with a pre-defined list of dynamic variables, which you can find here. These variables can be used through the {{$variableName}} notation. These are the ones that I’ve used:
- $guid: generates a random GUID (uuid-v4 style)
- $isoTimestamp: the current UTC ISO timestamp
- $randomDepartment: a random commerce category
Random values from lists
In many cases, you want to control the lists from which you pick the values. This can be done by leveraging the Pre-request Script tab, which allows you to write JavaScript.
pm.variables.set('customer', `${_.sample(["Noest", "Microsoft", "Your Azure Coach"])}`); pm.variables.set('type', `${_.sample(["External", "Internal"])}`);
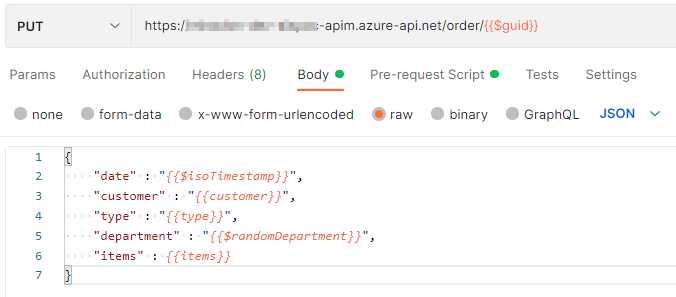
Dynamic JSON array
The number of items within my order should also be random. This can be achieved again through JavaScript in the Pre-request Script tab. Remark that I am also accessing dynamic values ($randomProduct) within this code sample.
let items = [] for (let i = 0; i < _.random(1,6); i++) { let item = { "product": '{{$randomProduct}}' , "amount": _.random(123) }; items[i] = item; } pm.variables.set('items', JSON.stringify(items))![]()
Execute multiple requests
In order to let Postman execute the dynamic request multiple times, you have to save it into a Postman collection. Click on the collection and choose Run. You can now choose the API requests that you want to run and the number of iterations. Bear in mind that the execution is sequential, not in parallel.
Conclusion
A simple solution for a simple challenge, so I am happy 🙂
Cheers
Toon